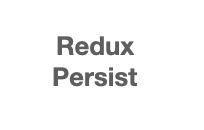
Redux-persist
미음제
·2022. 12. 2. 18:41
지난 포스트에서 Redux 예제를 사용하며 localStorage에 state를 저장해 사용하는 방법을 다뤘다. Redux persist 라이브러리를 통해 Web Storage에 state를 저장할 수 있다는 것을 알게 되어 직접 적용해보고 간단하게 정리한 내용을 작성하려고 한다.
Redux-persist
https://github.com/rt2zz/redux-persist#quickstart
GitHub - rt2zz/redux-persist: persist and rehydrate a redux store
persist and rehydrate a redux store. Contribute to rt2zz/redux-persist development by creating an account on GitHub.
github.com
애플리케이션에서 prop drilling을 회피하거나, state를 중앙화 하여 관리하기 위해 Redux를 사용한다. 그러나 전역 store에 저장한 데이터는 새로고침 혹은 브라우저를 종료하는 순간 초기화 된다.
이를 해결하기 위해 Web Storage에 저장해 사용한다.
Web Storage(localStorage, sessionStorage, cookie)
2주 전, K사 코딩 테스트를 응시하며 React Redux를 사용해야 했다. Redux를 공부했을 때 간단한 예제 정도만 구현해보고, 프로젝트를 할 때도 직접적으로 Redux를 사용하지 않아서 사용 방법을 까먹고
mieumje.tistory.com
Redux-persist 라이브러리는 persistReducer에 특정 Reducer를 결합하면, Web Storage에 state를 저장하거나 저장된 state를 가져올 수 있다.
기본 사용 법
// store/index.js
import { combineReducers, createStore } from "redux";
import { counterReducer } from "./reducer/counter";
import { taskReducer } from "./reducer/tasks";
import { persistReducer } from "redux-persist";
import storage from "redux-persist/lib/storage";
const persistConfig = {
key: "task",
storage,
};
export const rootStore = combineReducers({
counterReducer,
taskReducer: persistReducer(persistConfig, taskReducer),
});
export const store = createStore(rootStore);
Web Storage에 저장하고 싶은 state를 변경하는 Reducer를 persistReducer로 묶어 사용한다. config 객체와 Reducer를 인자로 받는다.
persistConfig
해당 객체의 key 값을 통해 Reducer에 등록하게 된다. key 값은 Web Storage에 저장되는 key 값으로 활용된다.
persistConfig.storage
Web Storage 중 사용할 storage를 지정한다.
- import storage from 'redux-persist/lib/storage' : localStroage
- import storageSession from 'redux-persist/lib/storage/session' : sessionStorage
persistConfig.whitelist or blacklist
배열 형태로 reducer를 전달(reducer 이름을 string으로)한다.
- whitelist : 사용하고 싶은 reducer만 storage에 저장
- blacklist : 제외할 reducer
PersistGate
// root index.js
import React from "react";
import ReactDOM from "react-dom/client";
import "./index.css";
import App from "./App";
import reportWebVitals from "./reportWebVitals";
import { Provider } from "react-redux";
import { persistStore } from "redux-persist";
import { PersistGate } from "redux-persist/integration/react";
import { store } from "./store";
const persistor = persistStore(store);
const root = ReactDOM.createRoot(document.getElementById("root"));
root.render(
<React.StrictMode>
<PersistGate loading={null} persistor={persistor}>
<Provider store={store}>
<App />
</Provider>
</PersistGate>
</React.StrictMode>
);
reportWebVitals();
PersistGate는 전역 store를 전달하기 위한 Redux의 Provider처럼 persistor를 전달하는 역할이다. persistConfig 객체에 정의한 key 값으로 Reducer를 등록한다.
persisted state가 반영되기까지 loading prop으로 전달한 React instance를 노출할 수 있다. 해당 prop 값은 null 값을 지정할 수 있다.
Persist Store
Web Storage에 저장하고 싶은 redux store를 인자로 넘겨준다. persistStore 메소드는 persistor 오브젝트를 return 한다.
persistor object
해당 오브젝트는 persistStore에 의해 반환된 오브젝트로 아래 메소드를 포함하고 있다. Reducer에 존재하는 동작 외에 특정 기능을 수행할 수 있는 메소드들이다.
- .purge() → action을 dispatch 하여 Storage에 저장된 데이터를 삭제하고, 다른 값으로 초기화한다.
- .flush() → state를 disk에 저장한다.
- .pause() → persist state를 storage에 저장하는 것을 중단한다.
- .persist() → pause()와 반대되는 것으로, storage에 저장하는 것을 재개한다.
참고
Redux Persist를 소개합니다.
Redux의 State값을 local storage에 저장하기 쉽게 도와주는 라이브러리입니다.
haragoo30.medium.com
Redux Persist 알아보기
Redux와 Redux Persist를 사용하여 앱을 종료해도 지속되는 Store 만들기
medium.com
'Developer > React' 카테고리의 다른 글
[React] 이미지 업로드 미리보기(FileReader / createObjectURL) (1) | 2022.09.05 |
---|